Follow these steps to display selected row data from a GridView in TextBox controls within an ASP.NET MVC application. This example will show you how to create a simple GridView, choose a row, and populate TextBox controls with data from that row.
Step 1: Set Up Your ASP.NET MVC Project
Open Visual Studio.
Create a new ASP.NET MVC project:
Go to File > New > Project.
Select ASP.NET Web Application and name your project (e.g., GridViewToTextBoxApp).
Choose the MVC template and click OK.
Step 2: Create the Model
Create a model class that represents the data you want to display in the GridView.
Create a new class in the Models folder named User.cs:
csharp
public class User
{
public int Id { get; set; }
public string Name { get; set; }
public string Email { get; set; }
}
Step 3: Create the Controller
Create a new controller named HomeController.cs:
csharp
using System.Collections.Generic;
using System.Web.Mvc;
using YourNamespace.Models; // Update with your actual namespace
public class HomeController : Controller
{
// Sample data to simulate a database
private List<User> users = new List<User>
{
new User { Id = 1, Name = “John Doe”, Email = “john@example.com” },
new User { Id = 2, Name = “Jane Smith”, Email = “jane@example.com” },
new User { Id = 3, Name = “Sam Brown”, Email = “sam@example.com” }
};
public ActionResult Index()
{
return View(users);
}
[HttpPost]
public ActionResult GetUserDetails(int id)
{
var user = users.Find(u => u.Id == id);
return Json(user);
}
}
Step 4: Create the Index View
Create the Index view for the HomeController:
Right-click on the Index action and select Add View.
Name the view Index.cshtml.
xml
@model IEnumerable<YourNamespace.Models.User> // Update with your actual namespace
@{
ViewBag.Title = “User List”;
}
<h2>User List</h2>
<table id=”userTable” class=”table”>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Email</th>
<th>Select</th>
</tr>
</thead>
<tbody>
@foreach (var user in Model)
{
<tr>
<td>@user.Id</td>
<td>@user.Name</td>
<td>@user.Email</td>
<td>
<button class=”select-user” data-id=”@user.Id”>Select</button>
</td>
</tr>
}
</tbody>
</table>
<h3>User Details</h3>
<div>
<label>ID:</label>
<input type=”text” id=”userId” readonly />
</div>
<div>
<label>Name:</label>
<input type=”text” id=”userName” />
</div>
<div>
<label>Email:</label>
<input type=”text” id=”userEmail” />
</div>
<script src=”https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js”></script>
<script>
$(document).ready(function () {
$(‘.select-user’).click(function () {
var userId = $(this).data(‘id’);
$.ajax({
url: ‘@Url.Action(“GetUserDetails”, “Home”)’,
type: ‘POST’,
data: { id: userId },
success: function (data) {
$(‘userId’).val(data.Id);
$(‘userName’).val(data.Name);
$(‘userEmail’).val(data.Email);
}
});
});
});
</script>
Step 5: Run Your Application
Start the application by pressing F5.
Navigate to the home page. You should see a table of users.
Click the “Select” button next to any user. The user’s details will populate the TextBox controls below the table.
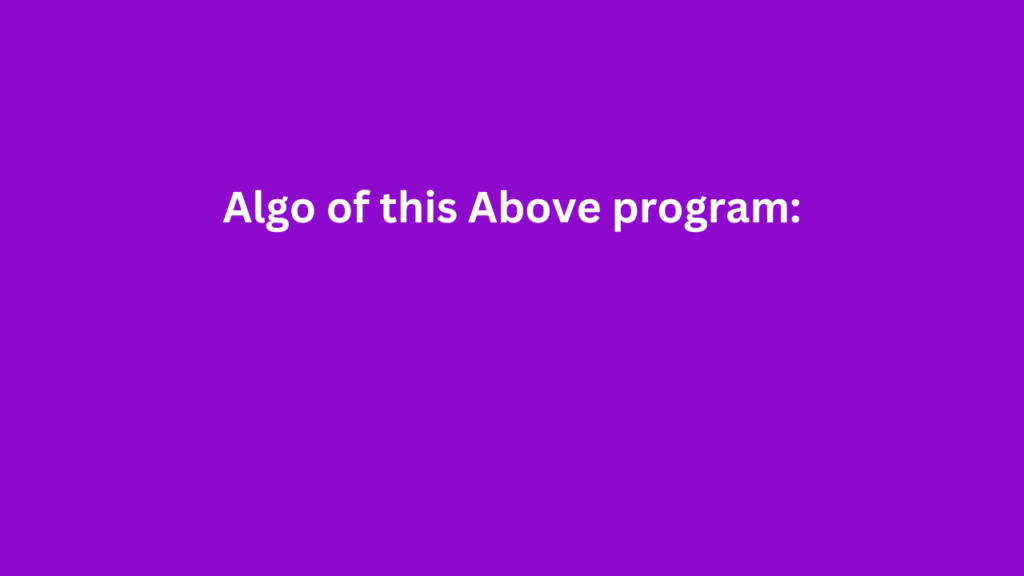
Central Topic: Display Selected Row Data
Sub-branches:
- View (cshtml):
- Define TextBoxes:
- Include HTML elements for TextBoxes where selected data will be displayed.
- Assign unique IDs for each TextBox.
- Implement GridView with Selection:
- Use
@Html.GridView
helper to render the GridView. - Set
AllowSelection
property totrue
. - Optionally, define a selection mode (single or multiple).
- Use
- Event Handling (Optional):
- Include JavaScript code to handle row selection (if not using server-side event).
- Capture selected row index or data.
- Trigger an AJAX call to the controller with selected data (if desired).
- Include JavaScript code to handle row selection (if not using server-side event).
- Define TextBoxes:
- Controller:
- Define Action Method:
- Create an action method to handle the request for displaying selected data.
- This method can receive selected data as parameters. (from view or server-side event)
- Retrieve Data (Optional):
- If data is not passed from the view, retrieve it from the model or database based on selection.
- Prepare View Model:
- Create a view model object to hold the selected data.
- Populate the view model with data from the selected row.
- Define Action Method:
- Model (Optional):
- Define Properties:
- Define properties in your model class corresponding to the data displayed in the GridView.
- Define Properties:
- Event Handling (Server-side):
- SelectedIndexChanged Event (Optional):
- Handle the
SelectedIndexChanged
event of the GridView (if not using JavaScript). - Access the selected row data within the event handler.
- Redirect to a different action method with selected data (or update view model directly).
- Handle the
- SelectedIndexChanged Event (Optional):
Additional Considerations:
- Data Binding:
- Use data binding syntax (@Model.<property>) to bind selected data from the view model to the TextBoxes in the View.
- Error Handling:
- Implement error handling for scenarios where no row is selected or data retrieval fails.
- Security:
- Validate user input and sanitize data before processing to prevent security vulnerabilities.
Benefits:
- Simplifies data editing and visualization.
- Improves user experience by pre-populating forms with selected data.
Tools:
- Visual Studio (or any IDE for ASP.Net MVC development)
- HTML editor
Note: This mindmap provides a general overview. Specific implementation details may vary depending on your project requirements and chosen approach.
Summary
This tutorial shows you how to display chosen row data from a GridView (simulated with an HTML table) into TextBox controls in an ASP.NET MVC application. The approach entails developing a model, a controller with sample data, and a view that uses jQuery for AJAX requests to retrieve and display user information based on the selected row. You can improve this implementation by connecting it to a real database and including error handling.